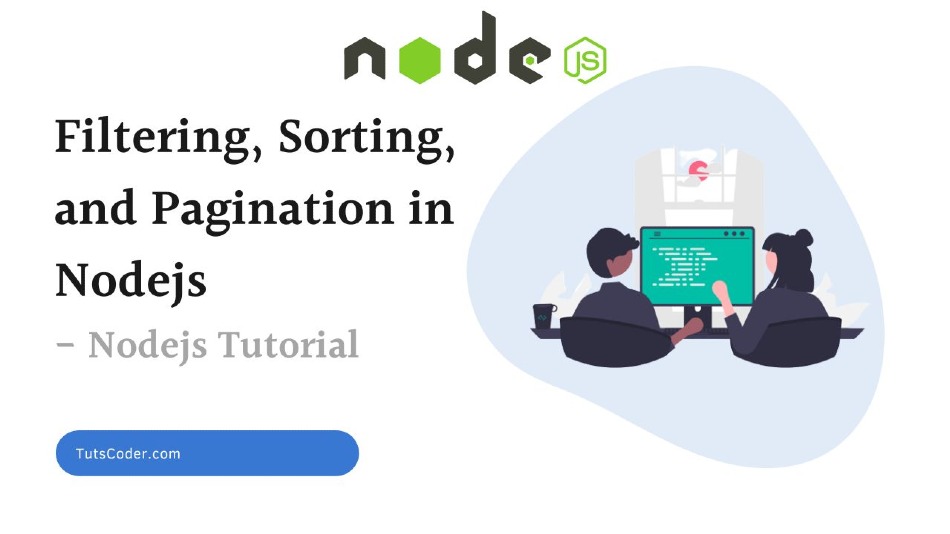
Filtering, Sorting, and Pagination in Nodejs
In this article, we will see how to do filtering, sorting, limiting fields, and pagination in nodejs with express.js and mongoose.
Filtering
Case1: Suppose you want to get a blog article, which has an active flag set to true and the category is 'Angular', so in this scenario, we can do this, as below:
Sample Url: https://www.example.com/posts?active=true&category=angular
const queryObj = { ...req.query };
const excludedFields = ['page', 'sort', 'limit', 'fields'];
excludedFields.forEach(el => delete queryObj[el]);
const posts = await Post.find(queryObj);
Above first we have made clone of object and exscluded some query params from query obj to later uses.
Now let's make it advance and add another feature like filtering with less then, greater then value, for instacne in our example we need posts whose views are greater then 1000, let's see how we can do it:
Sample URL : https://www.example.com/posts?views[gte]=1000
const queryObj = { ...req.query };
const excludedFields = ['page', 'sort', 'limit', 'fields'];
excludedFields.forEach(el => delete queryObj[el]);
// Advanced filtering
let queryStr = JSON.stringify(queryObj);
//Replaced string gte to $gte
queryStr = queryStr.replace(/\b(gte|gt|lte|lt)\b/g, match => `$${match}`);
const result = await Post.find(JSON.parse(queryStr));
Sorting
Now suppose we want to sort our blog post article by specifc criteora or default to created date, let's do it :
sample URL: https://www.example.com/posts?sort=views
if (req.query.sort) {
// if there is multiple sort option, replace (,)comma with space
const sortBy = req.query.sort.split(',').join(' ');
this.query = this.query.sort(sortBy);
} else {
this.query = this.query.sort('-createdAt');
}
In above example first, we will check is there any specifc recommnadaton for sorting? if not then default sort by created date at decending order.
Limiting Fields
There is alway good prtatice to send only specifc and required fileds to client side, instead of sending whole objetc from database,we can do that using:
Sample URL: https://www.example.com/posts?fields=name,author,slug
if (req.query.sort) {
const fields = req.query.sort.fields.split(',').join(' ');
this.query = this.query.select(fields);
} else {
//remove default __v fields from result
this.query = this.query.select('-__v');
}
Pagination
Sample Url: https://www.example.com/posts?page=2&limit=10
const page = this.queryString.page * 1 || 1;
const limit = this.queryString.limit * 1 || 100;
const skip = (page - 1) * limit;
this.query = this.query.skip(skip).limit(limit);