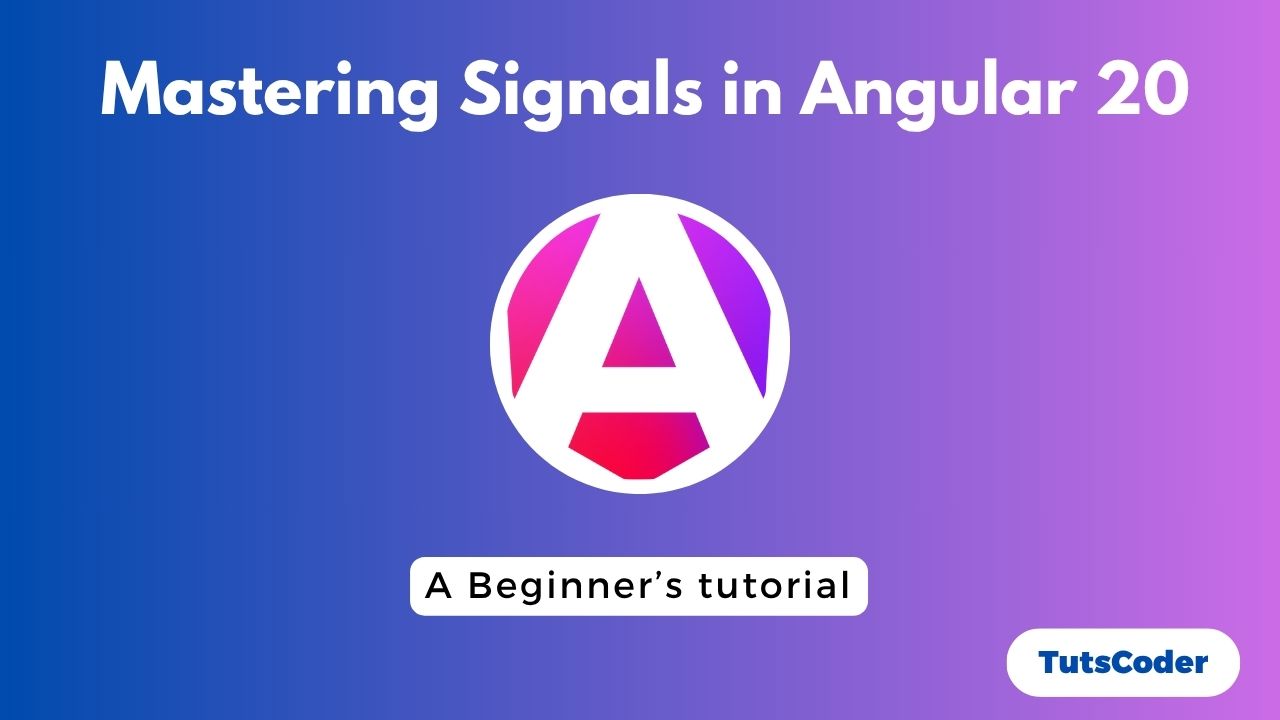
Mastering Angular Signals in Angular 20+: A Beginner’s Guide
Angular’s evolution continues to surprise developers with powerful tools, and in Angular 18+, one of the most exciting additions is Signals. If you're new to Signals or reactive programming, this guide will walk you through setting up Signals, understanding common use cases, and discovering why Signals stand out compared to other reactive patterns.
Table of Contents
- What Are Signals in Angular?
- Setting Up Signals in Angular 18+
- Real-Life Example: Signals in Action
- Common Use Cases for Signals
- Benefits of Signals Over Other Reactive Patterns
- Transitioning from Observables to Signals: A Comparison
- Quick Tips for Using Signals Effectively
- Wrapping Up: Why Signals Are a Game-Changer for Angular Developers
What Are Signals in Angular?
Simply put, Signals are a reactive mechanism for handling state changes. They introduce a more efficient way of tracking and updating application data without relying on the traditional @Input
or @Output
properties. Signals can detect changes automatically, making them a robust tool for updating your UI without additional boilerplate code.
How Signals Work?
Think of Signals as an automatic notification system. 🎯 Imagine a smart assistant that knows when and where something has changed in your application. Signals do just that! When data updates, any UI elements bound to that data will reflect the change without additional coding. This mechanism cuts down on the complexity that often comes with tracking and responding to data changes in Angular apps.
Check it out, Official Angular Signals documentation
Setting Up Signals in Angular 18+
Before diving into Signals, make sure your project is updated to Angular 18+ (you can update by running ng update
if needed). Once your app is up-to-date, follow these steps to set up and use Signals:
Import Signals: First, import Signals in the component where you plan to use them.
import { signal } from '@angular/signals';
Define a Signal: Declare a Signal by calling signal
and passing it an initial value.
export class AppComponent {
count = signal(0);
}
Update Signal Values: Signals are reactive, meaning the UI automatically updates when the Signal value changes. You can update a Signal like this:
this.count.set(this.count() + 1); // Increments count by 1
Use Signals in the Template: To display the Signal value, simply bind it in your template.
<h1>Counter: {{ count() }}</h1>
<button (click)="increment()">Increment</button>
Real-Life Example: Signals in Action
Imagine you’re building a shopping cart. As a user adds items, you want the cart’s total to update automatically. Here’s how Signals make it easy:
// In the component
export class ShoppingCartComponent {
cartTotal = signal(0);
addItem(price: number) {
this.cartTotal.set(this.cartTotal() + price);
}
}
In the template, you simply display the cart total:
<p>Your Cart Total: ${{ cartTotal() }}</p>
<button (click)="addItem(10)">Add $10 Item</button>
Each time addItem
is called, cartTotal
updates, and the UI displays the latest total. No need for complex change detection—Signals handle it for you! 🎉
Read, Understanding the Difference between Constructor and ngOnInit in Angular
Common Use Cases for Signals
Signals shine in scenarios where dynamic, real-time updates are essential. Here are a few practical use cases:
- Forms with Real-Time Validation: Signals can be used to track input changes and immediately display validation messages.
- Live Counters and Timers: For apps requiring counters (like a step tracker or countdown timer), Signals make it easy to auto-update the display.
- Data Filtering in Real Time: Signals simplify implementing search and filter functions that react to user input instantly.
Benefits of Signals Over Other Reactive Patterns
Angular developers are familiar with Observables
and BehaviorSubject
for handling asynchronous data, but Signals offer unique advantages:
- Automatic Tracking: Signals automatically track dependencies, reducing the need for manual subscriptions and unsubscribing. Say goodbye to potential memory leaks! 👋
- Simplified Code: Using Signals reduces the boilerplate code typically associated with Observables, resulting in cleaner, easier-to-read code.
- Performance: Signals are optimized for minimal computation, so the application performs faster, even with frequent data changes.
Transitioning from Observables to Signals: A Comparison
Let’s compare how Signals simplify state handling versus using Observables:
Using Observables
With Observables, you’d typically write a lot of boilerplate code:
cartTotal$ = new BehaviorSubject(0);
addItem(price: number) {
this.cartTotal$.next(this.cartTotal$.getValue() + price);
}
Using Signals
In Signals, there’s no need for .subscribe()
or .next()
:
cartTotal = signal(0);
addItem(price: number) {
this.cartTotal.set(this.cartTotal() + price);
}
In most cases, Signals require less code and automatically manage state updates without you having to worry about subscribing and unsubscribing from changes.
Quick Tips for Using Signals Effectively
- Use Signals for Local State: When the state only affects a specific component or element, Signals are ideal.
- Avoid Signals for Heavy Asynchronous Work: For network requests or streams of data, Observables are still better suited due to their async capabilities.
- Combine with Angular’s Built-In Directives: Signals work seamlessly with ngIf, ngFor, and other structural directives, making it easy to build dynamic UIs.
Wrapping Up: Why Signals Are a Game-Changer for Angular Developers
With Signals, Angular 18+ has opened up a whole new way to handle reactive programming, making applications more responsive and the code easier to maintain. By adopting Signals, you can simplify state management, boost performance, and focus more on building features that matter to your users.
So go ahead—dive into Signals and see how this powerful tool can bring your Angular app to life!
Get Professional Angular Support
Need expert support for your Angular project? I'm available on Upwork and Fiverr to help you build, maintain, and enhance your applications with modern Angular practices.