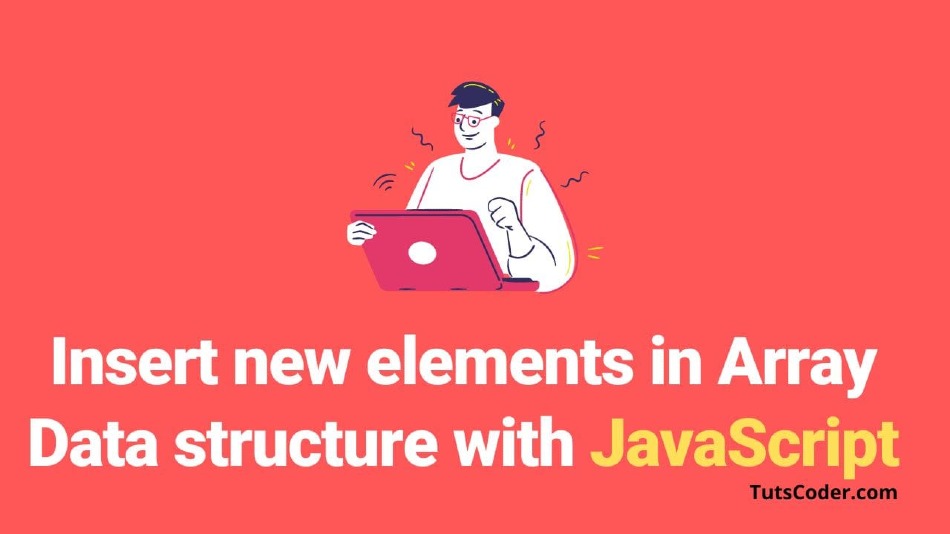
Insert new elements in Array Data structure with JavaScript
In this tutorial, we will learn how to insert the new elements in the array of data structures and algorithms with javaScript.
Insert new element in array:
let arr = [58, 40, 60, 65, 50];
let newEl = 30;
let position = 3;
for (let i = arr.length - 1; i >= 0; i--) {
if (i >= position) {
arr[i + 1] = arr[i];
if (i === position) {
arr[i] = newEl;
}
}
}
console.log(arr);
Same thing we can do using the Splice method like below:
arr.splice(position,0,newEl);