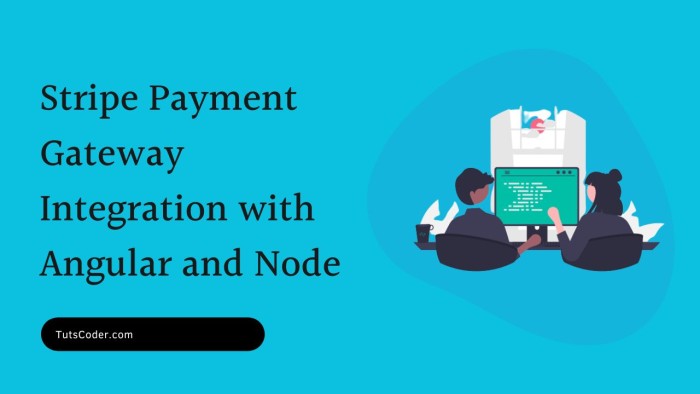
Implementing stripe with Node.js and Angular: A Comprehensive Guidepe Payment Gate
Integrating a payment gateway into your web application is crucial for handling transactions efficiently. Stripe, a popular payment processing platform, provides robust solutions for various payment needs. In this guide, we'll walk you through implementing Stripe payment gateway with Node.js and Angular, covering both backend and frontend integration.
Overview of Stripe Integration
Stripe offers a powerful API to integrate payment processing into your applications. Whether you're building an eCommerce platform, a subscription service, or a donation site, Stripe simplifies the payment process and ensures secure transactions. For a deeper understanding of Stripe’s features, you can refer to the official Stripe documentation.
Setting Up Stripe in Node.js
First, you need to set up Stripe in your Node.js application. Follow these steps to configure Stripe and create a checkout endpoint:
1. Install Stripe SDK
Install the Stripe Node.js SDK via npm:
npm install stripe
2. Configure Stripe
In your Node.js application, configure Stripe with your secret key:
const stripe = require('stripe')(process.env.STRIPE_SECRET);
3. Create the Checkout Endpoint
Add the following code to handle Stripe payments and update user data:
exports.makeStripeCheckout = async (req, res) => {
try {
const { scriptId } = req.body.token;
const userId = req.user._id;
const token = req.body.token;
// Create a customer
const customer = await stripe.customers.create({
email: "jigneshkumar1494@gmail.com",
source: token.id
});
// Create a charge
const charge = await stripe.charges.create({
amount: 1000,
description: "sample",
currency: "USD",
customer: customer.id
});
// Update the user's purchased scripts
const updated = await User.findByIdAndUpdate(
userId,
{ $addToSet: { purchasedScripts: scriptId } },
{ new: true }
);
res.json({
success: true,
message: 'Product purchased successfully.'
});
} catch (err) {
console.log(err);
res.status(500).json({
error: "failure"
});
}
};
For more on handling payments with Stripe, check out Stripe’s payment flow overview.
Implementing Stripe in Angular
To integrate Stripe on the frontend with Angular, follow these steps:
1. Add Stripe Checkout Script
Ensure the Stripe Checkout script is loaded:
invokeStripe() {
if (this.platformService.isBrowser()) {
if (!window.document.getElementById('stripe-script')) {
const script = window.document.createElement('script');
script.id = 'stripe-script';
script.type = 'text/javascript';
script.src = 'https://checkout.stripe.com/checkout.js';
script.onload = () => {
this.paymentHandler = (window).StripeCheckout.configure({
key: 'pk_test_LFQKUb2geFOlsg7uZxsDPplt',
locale: 'auto',
token: function (stripeToken: any) {
this.toastr.success('success', 'Payment has been successful!');
},
});
};
window.document.body.appendChild(script);
}
}
}
2. Implement the Payment Function
Create a function to handle Stripe payments:
buywithStripe(product: any) {
const amount = product.offerPrice ? product.offerPrice : product.price;
if (this.authService.isLoggedIn) {
const paymentHandler = (window).StripeCheckout.configure({
key: 'pk_test_LFQKUb2geFOlsg7uZxsDPplt',
locale: 'auto',
token: function (stripeToken: any) {
stripeToken.scriptId = this.scriptId;
this.paymentGetwaysService.makePayment(stripeToken).subscribe((response) => {
if (response.success) {
this.sweetAlertService.showSuccessMessage('Success', response.message);
this.router.navigate(['/dashboard']);
} else {
this.sweetAlertService.showWarningMessage('Warning', response.message);
}
});
},
});
paymentHandler.open({
name: 'TutsCoder',
description: 'Code Script',
amount: amount * 100,
});
} else {
this.router.navigate(['/auth/login']);
}
}
Handling Stripe Payments
Once you've integrated Stripe on both backend and frontend, ensure proper error handling and user feedback. Testing your implementation thoroughly will help catch any issues early on.
Testing Your Integration
Before going live, test your payment gateway integration extensively. Use Stripe's test mode to simulate transactions and verify that your application handles different scenarios correctly.
Conclusion
Integrating Stripe with Node.js and Angular can streamline your payment processing and enhance user experience. By following the steps outlined in this guide, you'll be able to implement a secure and efficient payment system for your web application.