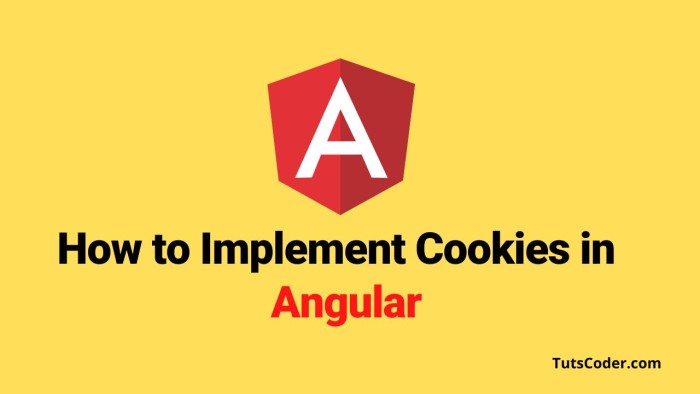
How to Implement Cookies in Angular 18: A Comprehensive Guide
Managing cookies is essential in web development, especially for creating a personalized and secure user experience. In Angular 18, you can easily work with cookies using the ngx-cookie-service
pluginโa powerful tool to help you handle cookies with ease. This guide will walk you through setting up cookies in your Angular application, focusing on the ngx-cookie-service
library, and provide best practices along the way.
What Are Cookies, and Why Use Them? ๐ซ
Cookies are small pieces of data stored in a user's browser. They help you remember user preferences, track session data, and enhance user experience. In Angular, cookies can be incredibly useful for storing session information, managing login states, and even setting user preferences.
Here's a step-by-step guide on how to implement cookies in Angular 16:
Step 1: Setting Up ngx-cookie-service in Angular
Letโs start by adding the ngx-cookie-service
plugin to our Angular project.
1.1 Install ngx-cookie-service
First, install the ngx-cookie-service
package. Open your terminal and run:
npm install ngx-cookie-service
1.2 Import the Service in Your Angular Module
After installing the plugin, import it into your Angular module, typically app.module.ts
:
import { NgxCookieService } from 'ngx-cookie-service';
@NgModule({
providers: [NgxCookieService],
})
export class AppModule {}
Step 2: Using Cookies in Angular ๐
Now that ngx-cookie-service
is set up, letโs look at how to create, read, and delete cookies in your application.
2.1 Inject CookieService into Your Component or Service
In your component, inject the CookieService
so you can start using its methods:
import { Component, OnInit } from '@angular/core';
import { CookieService } from 'ngx-cookie-service';
@Component({
selector: 'app-cookie-demo',
templateUrl: './cookie-demo.component.html',
})
export class CookieDemoComponent implements OnInit {
constructor(private cookieService: CookieService) {}
ngOnInit(): void {
// Actions will go here
}
}
2.2 Setting a Cookie ๐ช
To set a cookie, use the set
method. This method requires a cookie name, value, and expiration date.
this.cookieService.set('user', 'JohnDoe', 1); // Sets cookie to expire in 1 day
To make your cookie more secure, you can add optional flags like path
, domain
, and secure
:
this.cookieService.set('user', 'JohnDoe', 1, '/', 'example.com', true, 'Lax');
2.3 Getting a Cookie ๐
To retrieve a cookie value, use the get
method. Hereโs how you can check for the user
cookie:
const userName = this.cookieService.get('user');
console.log(`User: ${userName}`);
2.4 Checking If a Cookie Exists ๐
You can check if a cookie exists with the check
method. This is useful if you want to run certain actions based on whether a cookie is present.
if (this.cookieService.check('user')) {
console.log('User cookie exists!');
}
2.5 Deleting a Cookie โ
To remove a cookie, use the delete
method. This is handy when users log out or if you need to clear user preferences.
this.cookieService.delete('user');
Alternatively, to delete all cookies in the current path, use:
this.cookieService.deleteAll();
Common Use Cases for Cookies in Angular ๐
Cookies are versatile and can be used in several ways:
- Session Management: Keep track of user sessions to avoid repeated logins.
- User Preferences: Store preferences like theme, language, or layout choices.
- Analytics and Tracking: Monitor site usage by storing cookies that track user activity. (Ensure user consent to comply with data privacy laws!)
Troubleshooting Cookie Issues ๐ ๏ธ
- Cookies Not Setting: Ensure cookies are not blocked by your browser. Also, verify that your domain and path are correctly configured.
- Unexpected Expiry: Check if your cookies have correct expiration times and secure flags.
- Cross-Domain Issues: Cross-domain cookies require explicit domain settings to work correctly.
For more detailed information, visit the official documentation for ngx-cookie-service
here.
Wrapping Up ๐
Implementing cookies in Angular 18 with ngx-cookie-service
is straightforward and efficient! With the right setup, you can provide a personalized experience for your users and securely manage sessions and preferences.
Using cookies responsibly is essential in modern web applications. By following best practices, you can ensure your Angular app provides both functionality and privacy for your users. Happy coding! ๐