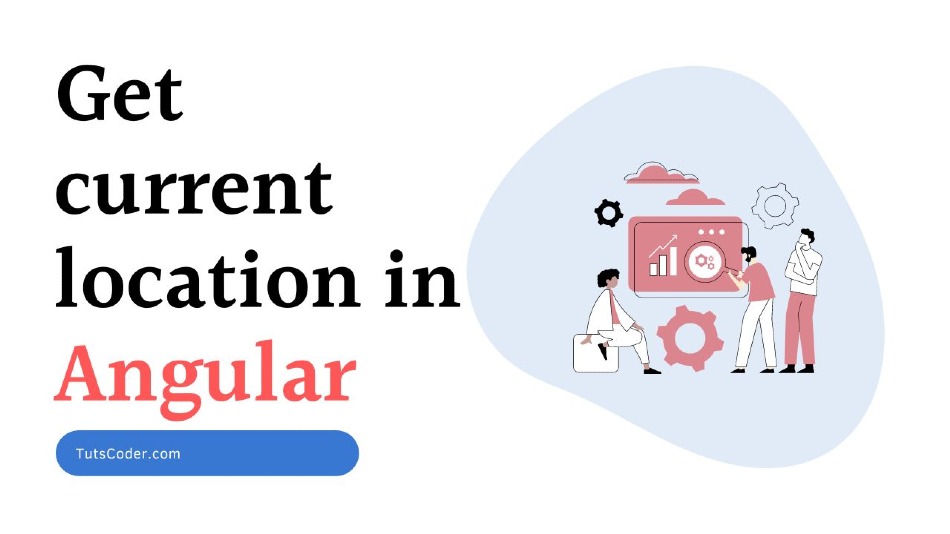
How to get current location in Angular 14+
In this article, we will see how to get the user's current location in Angular 14+.
Generate location service
ng g service services/location/location
It will create location service inside services folder
Now add below code in it
import { Injectable } from '@angular/core';
@Injectable({
providedIn: 'root',
})
export class LocationService {
constructor() {}
getCurrentLocation() {
return new Promise((resolve, reject) => {
if (navigator.geolocation) {
navigator.geolocation.getCurrentPosition(
(position) => {
if (position) {
console.log(
'Latitude: ' +
position.coords.latitude +
'Longitude: ' +
position.coords.longitude
);
let lat = position.coords.latitude;
let lng = position.coords.longitude;
const location = {
lat,
lng,
};
resolve(location);
}
},
(error) => console.log(error)
);
} else {
reject('Geolocation is not supported by this browser.');
}
});
}
}
Now use this location service in app.component.ts file as below:
const position: any = await this.locationService.getCurrentLocation();
console.log(position);
You will get the current latitude and longitude on the console.