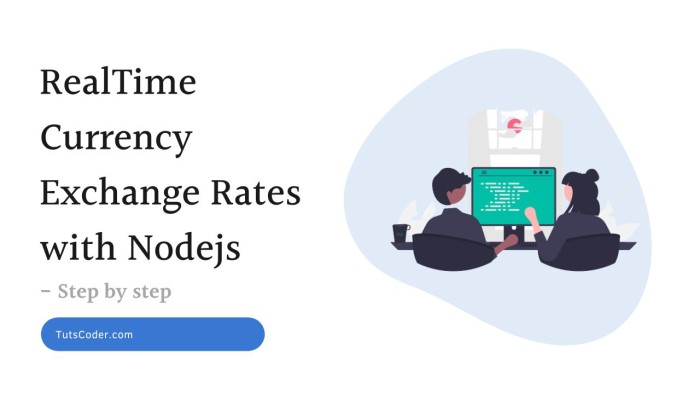
How to Fetch Real-Time Currency Exchange Rates in Node.js (With Bonus Caching Mechanism)
In today’s interconnected world, many applications rely on real-time currency exchange rates, whether for ecommerce, financial platforms, or travel services.
In this tutorial, we will walk you through how to fetch live currency exchange rates in a Node.js application using a third-party API.
Table of Contents
Why Fetch Real-Time Currency Exchange Rates?
Fetching up-to-date currency exchange rates is essential for apps handling international transactions. Whether you’re building an ecommerce platform that supports multiple currencies or a financial app that tracks exchange rates, real-time data ensures that users see the most accurate information.
However, making frequent API requests to fetch this data can increase costs and slow down your app, especially when the data is only updated periodically. To combat this, we’ll also introduce a caching mechanism that stores the fetched rates for a certain period, reducing the number of API requests and boosting your app’s performance.
Step 1: Setting Up the Node.js Environment
First, let’s set up a basic Node.js environment to fetch currency exchange rates. We’ll use the Axios
library to make HTTP requests and Moment.js
to handle time-related operations. If you haven’t installed them, you can do so by running:
npm install axios moment mongoose dotenv
Step 2: Fetching Currency Exchange Rates
For fetching real-time currency exchange rates, we’ll use a reliable API service. One such service is CurrencyAPI, which provides accurate exchange rate data. Sign up for an API key and include it in your .env
file for secure access.
Here’s how to fetch the exchange rate for Indian Rupees (INR) in a Node.js application:
const axios = require('axios');
const API_KEY = process.env.CURRENCY_API_KEY;
const API_URL = `https://api.currencyapi.com/v3/latest?apikey=${API_KEY}¤cies=INR`;
// Function to fetch the latest conversion rate
const fetchConversionRate = async () => {
try {
const response = await axios.get(API_URL);
const conversionRate = response.data.data.INR.value;
console.log(`The current exchange rate for INR is: ${conversionRate}`);
return conversionRate;
} catch (error) {
console.error('Error fetching conversion rate:', error);
throw new Error('Failed to fetch conversion rate');
}
};
module.exports = fetchConversionRate;
In this code:
- Axios is used to make a GET request to the API, fetching the latest exchange rate for INR.
- The API_URL contains the API endpoint, which includes your API key for authentication.
- The function fetches the exchange rate and returns the value for INR.
Step 3: Using the Fetch Function
You can call this function anywhere in your application to fetch the real-time exchange rate:
const fetchConversionRate = require('./utils/fetchConversionRate');
(async () => {
try {
const conversionRate = await fetchConversionRate();
console.log(`INR exchange rate: ${conversionRate}`);
} catch (error) {
console.error(error.message);
}
})();
This will fetch and log the latest exchange rate, ensuring your app is always updated with the most recent data.
Bonus: Implementing a Caching Mechanism for Efficiency
Fetching real-time exchange rates is great, but if you’re calling the API multiple times per day, you may end up paying more than necessary and introducing unnecessary latency. That’s where caching comes in handy.
We can implement a simple caching mechanism that stores the exchange rate in a database (like MongoDB) and only fetches new data if the cache is older than 24 hours. Here’s how to do it:
Step 1: Set Up MongoDB Model
First, define a Mongoose schema to store the currency exchange rate and the timestamp of when it was last updated:
const mongoose = require('mongoose');
const currencyRateSchema = new mongoose.Schema({
currency: { type: String, required: true },
rate: { type: Number, required: true },
updatedAt: { type: Date, default: Date.now },
});
module.exports = mongoose.model('CurrencyRate', currencyRateSchema);
This schema will store the currency code, exchange rate, and the time it was last updated.
Step 2: Modify the Fetching Function to Include Caching
We will now modify the original fetching function to check if a cached rate exists. If it’s less than 24 hours old, we’ll return the cached rate. Otherwise, we’ll fetch a new one and update the cache.
const axios = require('axios');
const moment = require('moment');
const CurrencyRate = require('../models/currencyRateModel');
const API_KEY = process.env.CURRENCY_API_KEY;
const API_URL = `https://api.currencyapi.com/v3/latest?apikey=${API_KEY}¤cies=INR`;
// Function to fetch and cache conversion rate
const getCachedConversionRate = async () => {
const currentTime = moment();
// Check if we already have a cached rate within the last 24 hours
let cachedRate = await CurrencyRate.findOne({ currency: 'INR' });
if (cachedRate && currentTime.diff(moment(cachedRate.updatedAt), 'hours') < 24) {
// If the rate is still valid, return the cached value
return cachedRate.rate;
}
// Otherwise, fetch the latest conversion rate from CurrencyAPI
try {
const response = await axios.get(API_URL);
const conversionRate = response.data.data.INR.value;
// Save the new rate to the database, updating the existing document
if (cachedRate) {
cachedRate.rate = conversionRate;
cachedRate.updatedAt = currentTime;
await cachedRate.save();
} else {
// If no rate exists, create a new document
cachedRate = new CurrencyRate({
currency: 'INR',
rate: conversionRate,
updatedAt: currentTime,
});
await cachedRate.save();
}
return conversionRate;
} catch (error) {
console.error('Error fetching conversion rate:', error);
throw new Error('Failed to fetch conversion rate');
}
};
module.exports = getCachedConversionRate;
Step 3: Using the Cached Rate
You can now replace the original fetch function with this new one that incorporates caching. Whenever you need the currency rate, it will either fetch from the cache or the API if the cached data is outdated:
const getCachedConversionRate = require('./utils/currencyConversion');
(async () => {
try {
const conversionRate = await getCachedConversionRate();
console.log(`INR exchange rate: ${conversionRate}`);
} catch (error) {
console.error(error.message);
}
})();
Benefits of Caching Exchange Rates
- Reduced API Costs: By caching exchange rates, you only make an API request once every 24 hours, saving on API usage fees.
- Faster Performance: Serving cached data from MongoDB is much faster than making an external API call, improving your app’s response time.
- Increased Reliability: If the API is down, you can still serve the cached exchange rates, ensuring your app remains functional.
Conclusion
Fetching real-time currency exchange rates in Node.js is a straightforward process, but integrating a caching mechanism adds an extra layer of efficiency and cost savings. By caching the data for 24 hours, you can reduce API costs, boost performance, and ensure that your app remains responsive even during API outages.