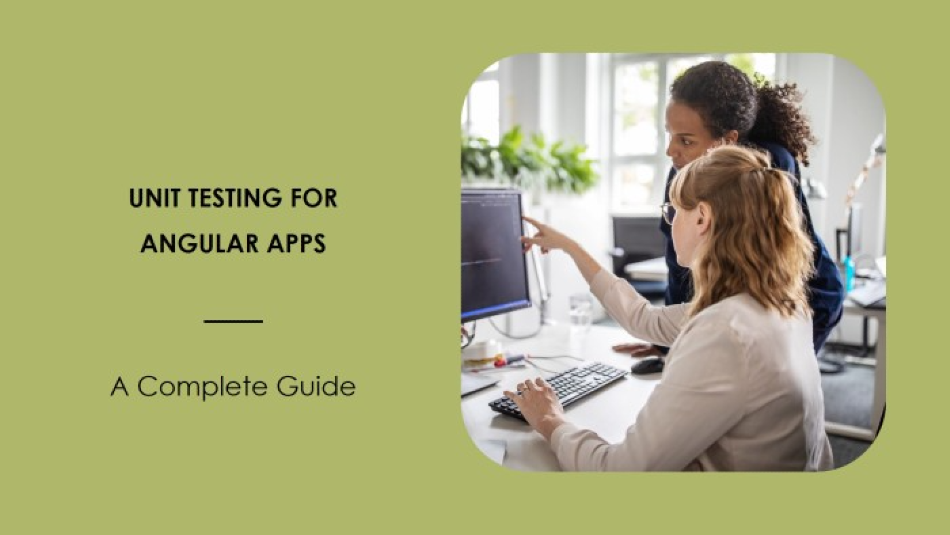
Complete Guide on Unit Testing for Angular Apps
Software testing, particularly unit testing, plays a significant role in the development of Angular apps. It makes sure that each separate part works properly on its own, which ultimately benefits software quality and development. It is particularly useful in Angular applications, where components and services are often interconnected and share information. This approach helps developers to detect and isolate the areas that require improvement, thus leading to the creation of a better quality application with higher maintainability.
Let’s help you to setup an Angular environment for unit testing, understand the tools frameworks involved and the best practices to enhance efficiency of the unit testing.
Preparation for Unit Testing in Angular
Testing in Angular is made easy by effective tools and frameworks that assist in testing your components or services. The first key step in process of unit testing is to prepare your testing environment correctly. Here's how you can prepare your Angular project:
- Angular CLI: To begin with, utilize Angular CLI to create a modern venture, which comes with Jasmine and Karma – the testing instruments that are coordinates into Angular.
- Jasmine: A testing system for Behavior-Driven Improvement in JavaScript.
- Karma: A test runner which enables you to run tests in real browsers.
- Initial Configuration: When you create a new project like ‘ng new my-project’ these tools will be setup and Angular CLI will also set up a basic test configuration.
- Running Tests: You can execute your tests on the ‘ng test’ command. This command stands for Karma test runner setup and starts running the tests in the browser with the updated result.
This setup does not only make it easier in the early stages, but it also comes with the added benefit of Angular’s ecosystem where you get to spend more time on writing proper tests instead of having to configure testing frameworks.
Understanding Angular Test Bed
The Angular Test Bed is an important aspect of Angular development kit that aims at helping developers when it comes to conducting some tests on the applications.
- Purpose of Angular Test Bed: The use of the test environment gives developers a representation of how individual components and services perform in a live Angular enviroment, thus serves well to give unit tests a realistic scenario.
- Controlled Testing Environment: One of the great features of Angular Test Bed is the ability to test in isolation; this is due to inability of the components and services to interfere with other components within the application. This isolation is very useful when identifying problems and checking for functionality.
- Ease of Setup: The Angular Test Bed does not need a lot of setup to be established. It makes the task easier by enabling the developers to understand what has to be tested rather than how to test.
- Flexibility: It provides the opportunity to test all possible aspects of an Angular app, ranging from separate components to specific services, giving a complete testing strategy.
- Reliability: Angular Test Bed is a tool that enables developers to work with the tests and get reliable outputs that are important in improving the performance of the applications.
Sub-Components Unit Tests
It is a best practice in Angular where components are tested to ensure that they work as intended across diverse scenarios.
- Importance of Testing Components:
Components testing keeps bugs at a manageable level during development, increases the quality of code, and ensures the proper functioning of an application in its growth.
- Key Aspects to Test in Angular Components:
- Component Creation and Initialization: Ensure that the component is created appropriately and initialized with the right data and its states. This helps to ensure that they are properly configured the first time they are utilized.
- Interaction Handling (Inputs and Outputs): Subsystems are usually interdependent. In testing inputs and yields, all the components receive and send the adjust information.
- Simulating User Interactions and Events: For the higher probability of the successful behavior of the component, it is necessary to imitate such actions as clicks, typing, or any navigation events. It assists in confirming the component’s runtime behaviour, including its functions of handling user interactions.
- Tips for Effective Component Testing:
- Isolate the Component: Perform some of the tests on components in isolation as this helps to identify those specific problems that originate from the specific component’s logic and architecture.
- Use Mock Data: Use mock data and services to understand how a part of the application works with various datasets and use cases.
- Automate Repetitive Tests: If you have tests that require running with different parameters or sequentially, use this mode to automate the process with no mistakes.
- Check Visual Changes: Ensure that state changes bring about the right changes in the user interface especially when dynamic classes or styles have been applied.
By concentrating on those areas, you can develop a powerful set of unit tests to support your Angular form and, in general, improve the quality of your application’s components.
Testing Services in Angular Applications
Angular Development Services are the ideal choice to manage data, perform business logic, and interact with backend servers. Testing of such services makes it possible to ensure that the basic functions of the application run smoothly.
- Role of Services in Angular Applications:
It is used to manage data and services for various components in the Angular application. These include operations like retrieving data from the servers, computations, and other processing requirements crucial for the functioning of the business application.
- Strategies for Testing Services:
- Isolation and Efficiency: When testing services it is necessary to separate them from other components and services in order not to overload test. This isolation assists in identifying specific problems that are unique to the functionality of the service.
- Mocking Dependencies: For isolating specific services, the use of mock libraries to mimic the responses of other services and or components is recommended. This ensures that you control the inputs and check the outputs without having to depend on other systems.
- Methods for Handling and Testing HTTP Requests: Services tend to rely on information obtained from HTTP requests. For mock testing of these services, you can employ the HttpClientTestingModule from the Angular platform. It allows you to set up your API to return a particular response based on certain data inputs and see how your service behaves.
By following these strategies, you will be able to effectively test your Angular services and make the application more stable. Testing services not only assist with keeping the applications up and running but also enhances the Angular application’s quality and efficiency.
Angular Unit Testing – Guidelines
Unit testing in Angular not only drives the quality of the code but also makes the development process optimal. The below-mentioned are some key best practices to follow:
Tips for Maintaining Effective Testing Strategies:
- Keep Tests Small and Focused: Every test should address one function or feature. It is easy to write, comprehend, and trace small tests rather than large ones.
- Test Behaviors Rather Than Implementations: Operate at a higher level of functionality by not emphasizing on the methods used in developing the code. This approach prevents having to switch tests when the implementation differs but the expected behavior is the same.
- Regular Use of Mocks and Spies: Use mocks and spies to pretend that different pieces are operating independently and uniquely in the system. This will ensure that the tests are not affected by other systems or complicated interactions within the system.
- Importance of Continuous Integration and Regular Test Runs: When testing is done as part of your development process, through tools such as continuous integration, it is easy to identify interferences. Using tests from the development phase helps ensure that improvements do not negatively affect other areas.
Complying with these practices ensure a reliable testing ground whereby any potential problems are easily detected thus making your Angular application more credible and sustainable.
Conclusion
It is essential to have unit tests while developing Angular applications as it helps to test each component and its integration with others. When unit testing is adopted as part of the development process, it helps to improve the stability of the application and also make updating easier.
Strong testing practices give a more stable and efficient end product with fewer bugs and therefore satisfied users. Stay tuned to the unit testing in your Angular projects to lay strong base for your applications so that when the time comes they are ready to face the real world challenges.