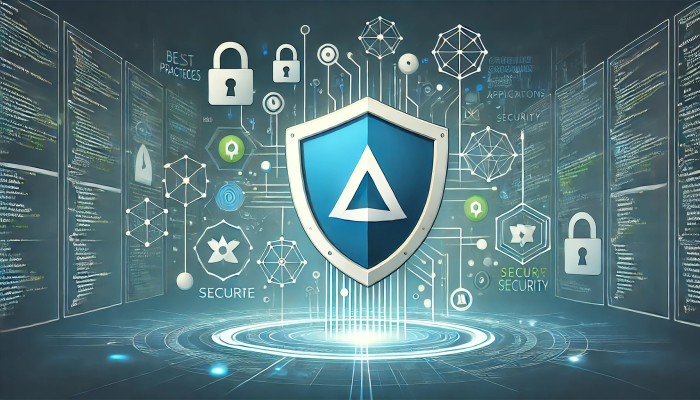
Top 7 Best Practices for Securing Your Angular Applications
Best Practices for Securing Angular Applications 🔐
When building with Angular, security is essential for keeping your application and users safe. In this guide, we’ll go over practical tips and examples to help you secure your Angular applications effectively. Whether you’re a beginner or a seasoned developer, these best practices can help protect your app from vulnerabilities. Let's dive in! 🚀
Why Security Matters in Angular Applications
Security vulnerabilities can lead to severe consequences, from data breaches to unauthorized access. Since Angular apps frequently handle sensitive data and run client-side, they can be prime targets for attacks. By following security best practices, you protect both your users and your app’s reputation.
1. Enable HTTP Security Headers 🛡️
HTTP headers are a powerful way to control app security. They let browsers know how to handle your data securely and protect against various attacks like XSS and clickjacking.
Key HTTP Headers for Security:
- Content Security Policy (CSP): This header restricts which resources (e.g., scripts, styles) are allowed to load. Only trusted sources should be listed here.
- X-Frame-Options: Prevents the app from being loaded in an iframe, which helps stop clickjacking.
- Strict-Transport-Security (HSTS): Forces the app to load over HTTPS, improving data confidentiality.
Example of Setting a CSP Header
In your server configuration, you can set CSP headers like so:
Content-Security-Policy: default-src 'self'; img-src https:;
2. Sanitize User Input 🧼
In Angular applications, user input is everywhere—forms, search bars, comments, and more. Unsanitized inputs can open the door to Cross-Site Scripting (XSS) attacks, where malicious scripts can run in your app.
Angular has built-in mechanisms for input sanitization, so always make use of Angular's DomSanitizer to clean potentially harmful data.
import { DomSanitizer, SafeHtml } from '@angular/platform-browser';
constructor(private sanitizer: DomSanitizer) {}
sanitizeContent(content: string): SafeHtml {
return this.sanitizer.bypassSecurityTrustHtml(content);
}
3. Use Angular’s Built-in Security Features 🛠️
Angular provides several built-in tools to help prevent security risks:
- Angular Security Contexts: These help prevent data from being rendered as HTML or JavaScript directly.
- Angular Interceptors: Add security headers automatically to each HTTP request.
- Router Guards: Control access to routes based on conditions, ideal for restricting certain areas of the app.
Example of Using a Router Guard
Router guards allow you to check authentication or permissions before granting route access.
canActivate(): boolean {
if (this.authService.isLoggedIn()) {
return true;
} else {
this.router.navigate(['/login']);
return false;
}
}
4. Avoid Storing Sensitive Data in Local Storage 🛑
Local storage may seem convenient, but it’s not secure for storing sensitive data like tokens or passwords. Instead:
- Use HTTP-only cookies to store session tokens. HTTP-only cookies cannot be accessed by JavaScript and are less vulnerable to attacks.
- For non-sensitive data, consider using Angular’s session storage.
5. Implement Role-based Access Control (RBAC) 🔑
RBAC restricts actions based on user roles. For example, admins can access admin settings, but regular users cannot. Implementing this in your Angular app enhances security by limiting who can access certain features.
Example of Role-based Access Control
canActivate(route: ActivatedRouteSnapshot): boolean {
const expectedRole = route.data.expectedRole;
const userRole = this.authService.getRole();
if (userRole === expectedRole) {
return true;
} else {
this.router.navigate(['/unauthorized']);
return false;
}
}
6. Regularly Update Dependencies 📦
Angular and its ecosystem are frequently updated, with many updates including security patches. Regularly updating your Angular packages, libraries, and dependencies keeps your application secure from known vulnerabilities.
Pro Tip: Use npm audit to Check for Vulnerabilities
The npm audit
command scans your dependencies for vulnerabilities and offers recommended fixes.
npm audit fix
7. Secure API Calls with Authentication and HTTPS 🌐
Any communication between your Angular app and backend should be secure. Follow these practices:
- Use HTTPS for all communication.
- Authenticate all API calls. OAuth 2.0, JWTs, and API keys are common options.
- Avoid exposing sensitive information in URLs.
Example: Interceptor to Add Auth Token
import { HttpInterceptor, HttpRequest, HttpHandler, HttpEvent } from '@angular/common/http';
import { Observable } from 'rxjs';
export class AuthInterceptor implements HttpInterceptor {
intercept(req: HttpRequest, next: HttpHandler): Observable> {
const authToken = this.authService.getToken();
const cloned = req.clone({
headers: req.headers.set('Authorization', `Bearer ${authToken}`)
});
return next.handle(cloned);
}
}
Final Thoughts on Securing Your Angular App 🔒
Security isn’t a one-time task. Regular audits, continuous updates, and following best practices are essential to keep your Angular applications safe. By prioritizing security, you can create a trustworthy and resilient app that stands strong against potential threats.
Key Takeaways 🎯
- Enable security headers like CSP, X-Frame-Options, and HSTS.
- Sanitize all user inputs and avoid storing sensitive data in local storage.
- Use Angular’s built-in tools like Router Guards and Security Contexts.
- Regularly update dependencies and secure your API communication.
Keeping these security best practices in mind helps create a safer experience for your users and strengthens your application’s integrity. Happy coding! 🎉